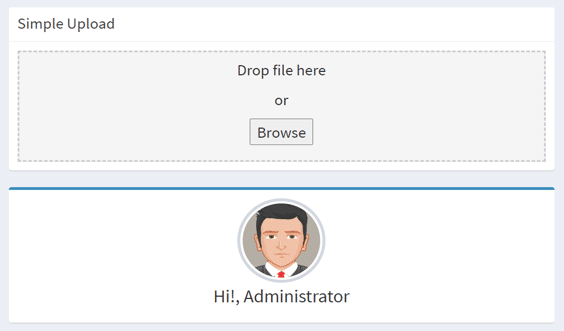
Contents
Hi, kali ini admin akan menulis mengenai cara upload file dan change avatar tanpa refresh halaman.
Contoh simple untuk mengupload banyak file menggunakan PHP jQuery. Dan untuk upload banyak file ini sangat berguna di mana Anda perlu mengunggah banyak file dalam aplikasi web.
Langkah 1
- Siapkan 2 file, v_upload.php dan submit.php
- Es teh manis dan pisang goreng 🙂
Langkah 2
- Download class upload => untuk mempermudah upload
- zebra_image => untuk resize gambar (jika diperlukan)
Langkah 3
Siapkan file v_upload.php dan buat kurang lebih seperti tampilan dibawah
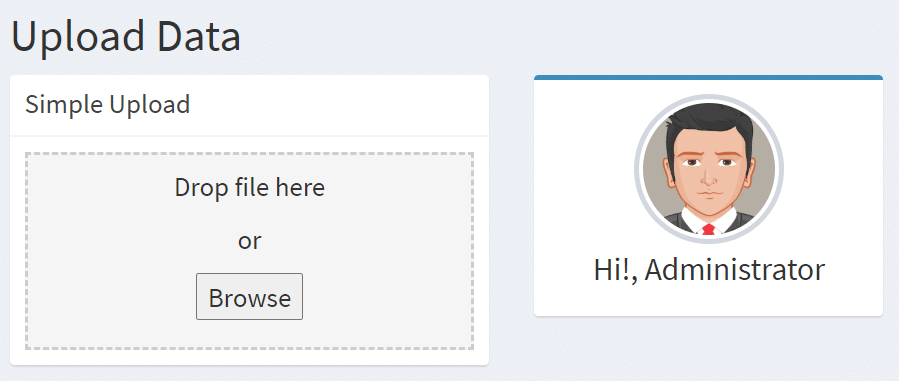
- Include jQuery library.
- CSS & HTML page with upload field.
- jquery/javascript code.
- PHP script to store the file.
<form method="POST" action="" class="form-horizontal" enctype="multipart/form-data">
<div id="drop_file_zone" ondrop="upload_file(event)" ondragover="return false">
<div id="drag_upload_file">
<p>Drop file here</p>
<p>or</p>
<p><input type="button" value="Browse" onclick="file_explorer();"></input></p>
<input type="file" id="selectfile" multiple></input>
</div>
</div>
<div id="isView_file"></div>
<input type="hidden" name="fileLain" id="fileLain" style="width:100%" value=""></input>
</form>
<form method="POST" action="" class="form-horizontal" enctype="multipart/form-data">
<div id="preview">
<input name="file" id="file" onchange="$(this).parent().parent();" style="display: none;" type="file"></input>
<img class="profile-user-img img-responsive img-circle hilang" src="<?php echo "assets/images/avatar.png"; ?>" alt="User profile picture" onclick="$(this).parent().find('input[type=file]').click();">
</div>
</form>
Tambahkan CSS supaya tampilan pada Simple upload seperti diatas
<style type="text/css">
#drop_file_zone {
background-color: #f5f5f5;
border: #ccc 2px dashed;
padding: 8px;
font-size: 18px;
}
#drag_upload_file {
width:100%;
margin:0 auto;
}
#drag_upload_file p {
text-align: center;
}
#drag_upload_file #selectfile {
display: none;
}
</style>
Tambahkan juga jquery / javascript seperti dibawah ini. Fungsi ini untuk post action supaya tidak refresh saat browse dan panggil submit.php
<script type="text/javascript">
// SIMPLE UPLOAD
var fileobj;
function upload_file(e) {
e.preventDefault();
ajax_file_upload(e.dataTransfer.files);
}
function file_explorer() {
document.getElementById('selectfile').click();
document.getElementById('selectfile').onchange = function() {
files = document.getElementById('selectfile').files;
ajax_file_upload(files);
};
}
function ajax_file_upload(file_obj) {
if(file_obj != undefined) {
var form_data = new FormData();
for(i=0; i<file_obj.length; i++) {
form_data.append('file[]', file_obj[i]);
}
$.ajax({
type: 'POST',
url: "submit.php?upload=sample1",
contentType: false,
processData: false,
data: form_data,
success:function(response) {
var convert = response.slice (0, -1);
$('#fileLain').val(convert);
$('#isView_file').html(convert.split(",").join("<br />"));
$('#selectfile').val('');
}
});
}
}
$(function() {
// CHANGE AVATAR IMAGE
$("#file").change(function() {
var fd = new FormData();
var files = $('#file')[0].files[0];
fd.append('file',files);
// AJAX request
$.ajax({
type: 'POST',
url: "submit.php",
data: fd,
contentType: false,
processData: false,
success: function(response){
if(response !== 'error'){
// Show image preview
$('#preview').html("<img class='profile-user-img img-responsive img-circle ada' src='file/"+response+"' alt=\"User profile picture\">");
$('.image').html("<img src=\"file/"+response+"\" class=\"img-circle\" alt=\"User Image\">");
}
else{
alert('Info : Please select a valid image file (JPEG/JPG/PNG).');
}
}
});
});
});
</script>
Langkah 4
Buat file submit.php, isinya seperti berikut
- require / include file class yang sudah di download tadi
- masukan function berikut untuk membantu filtering dan cek httprequest
- script request upload dan berikut file lengkapnya
<?php
require "../../../includes/upload.php";
require "../../../includes/zebra_image.php";
// AJAX request
function is_ajax()
{
return isset($_SERVER['HTTP_X_REQUESTED_WITH']) && strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest';
}
function filter($str)
{
$str = preg_replace('|%([a-fA-F0-9][a-fA-F0-9])|', '', $str);
$str = preg_replace('/[a-z0-9]+;/i','',$str);
$str = preg_replace('/\s+/', ' ', $str); // spasi lebih
$str = preg_replace('/[^%.,+&@#?$=()\/A-Za-z0-9 _-]/', '', $str);
return $str;
}
if(is_ajax())
{
if(isset($_REQUEST['upload']))
{
foreach($_FILES['file']['name'] as $key=>$val)
{
$file_name = $_FILES['file']['name'][$key];
$ext = strtolower(pathinfo($file_name, PATHINFO_EXTENSION));
$filenamewithoutextension = pathinfo($file_name, PATHINFO_FILENAME);
$filename_to_store = filter($filenamewithoutextension). '_' .uniqid(). '.' .$ext;
move_uploaded_file($_FILES['file']['tmp_name'][$key], getcwd(). '/file/'.$filename_to_store);
echo $filename_to_store.",";
}
}
else{
if (isset($_FILES['file']))
{
$allowedFile = array("jpeg", "jpg","png");
$upload = new Upload($_FILES['file'],"file/",1000000,$allowedFile);
$uploadedFile = $upload->GetResult()['path'];
$temporary = explode(".", $_FILES["file"]["name"]);
$file_extension = end($temporary);
$resize_image = new Zebra_Image();
$resize_image->source_path = getcwd(). 'file/'.$uploadedFile;
// indicate a target image
$resize_image->target_path = getcwd(). 'file/' . $uploadedFile;
// resize
// and if there is an error, show the error message
if (!$resize_image->resize(100, 100, ZEBRA_IMAGE_CROP_CENTER));
// from this moment on, work on the resized image
$resize_image->source_path = getcwd(). 'file/' . $uploadedFile;
$resize_image->preserve_aspect_ratio = true;
$resize_image->enlarge_smaller_images = true;
$resize_image->preserve_time = true;
}
if(!empty($_FILES))
{
try
{
## ALERT
if(!empty($_FILES["file"]["name"])){
if(in_array(strtolower($file_extension), $allowedFile))
{
/*
// Modify here for saving into database
$data = array();
if(!empty($uploadedFile)){
array_push($data['usr_img'] = $uploadedFile);
}
db->update();
*/
echo $uploadedFile;
}
else{
echo "error";
}
}
}
catch(Exception $e){
echo $e->getMessage();
}
}
else{
echo 'Error: fail upload data.';
}
}
}
Langkah 5
Jangan lupa siapkan folder “file” untuk menampung file-file hasil upload tersebut
Demikian tutorial untuk kali ini, di lanjutkan ke tutorial selanjutnya.
Author Profile
- Hi my name is Ricki, I am a blogger from Indonesia. Founder of erkamoo.com, Besides creating Web Applications, I also write about Blogging Tips and Tutorials on Programming, Databases, HTML.
Latest entries
Intro5 Juni 2025Berbagai Nama Anomali yang sedang Trend di Tiktok
Intro3 Januari 2025Manfaat Mindfulness untuk Kesehatan dan Produktivitas Pekerjaan di Tahun Baru
Tips & Trick3 Desember 202410 Rekomendasi Situs Download Game MOD (Terlengkap)
Tips & Trick3 November 202410 Emulator Game Android di PC dan Laptop yang Paling Digemari